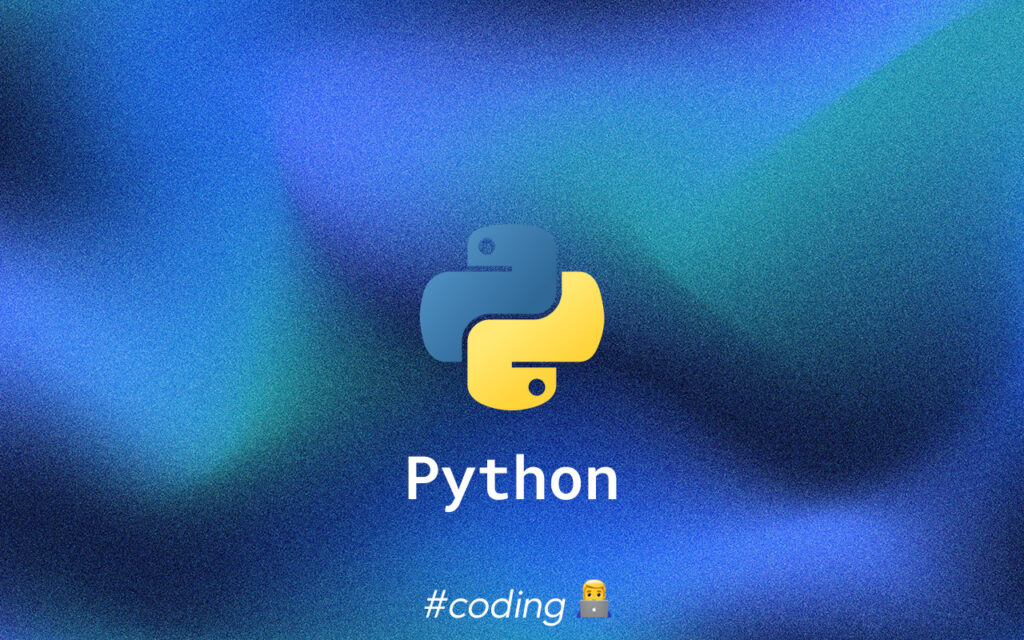
Python for Desktop Applications: Building Cross-Platform Apps with Kivy
In the ever-evolving world of software development, creating applications that work seamlessly across different operating systems is more important than ever. Enter Python and Kivy – a powerful combination that allows developers to build cross-platform desktop applications with ease. This article will guide you through the process of using Python and Kivy to create versatile, user-friendly desktop apps that run on Windows, macOS, and Linux.
Why Choose Python and Kivy for Desktop Development?
Before we dive into the how-to, let’s explore why Python and Kivy make such a great team for desktop application development:
- Python’s Simplicity: Python’s clean syntax and extensive libraries make it an ideal language for rapid development.
- Kivy’s Flexibility: Kivy is an open-source Python library for developing cross-platform applications with natural user interfaces.
- Cross-Platform Compatibility: Write once, run anywhere – Kivy apps work on Windows, macOS, Linux, iOS, and Android.
- Rich Set of Widgets: Kivy provides a wide range of customizable UI elements.
- Active Community: Both Python and Kivy have large, supportive communities, making problem-solving easier.
Getting Started with Kivy
To begin your journey with Kivy, you’ll need to set up your development environment:
- Install Python: If you haven’t already, download and install Python from python.org.
- Install Kivy: Use pip to install Kivy by running
pip install kivy
in your command prompt or terminal. - Choose an IDE: While any text editor will do, IDEs like PyCharm or Visual Studio Code offer helpful features for Python development.
Building Your First Kivy Application
Let’s create a simple “Hello, World!” application to get a feel for Kivy’s structure:
from kivy.app import App from kivy.uix.label import Label class HelloWorldApp(App): def build(self): return Label(text='Hello, World!') if __name__ == '__main__': HelloWorldApp().run()
This basic script creates a window with a label displaying “Hello, World!”. It demonstrates the fundamental structure of a Kivy application:
- Importing necessary Kivy modules
- Creating an
App
class - Defining the
build()
method to return the root widget - Running the application
Designing User Interfaces with Kivy Language
While you can create UIs programmatically, Kivy provides its own domain-specific language for UI design called Kivy Language (KV Language). This separates the logic from the presentation, making your code more maintainable:
#:kivy 1.0 <HelloWorld>: Label: text: 'Hello, World!' font_size: 30 center: root.center
This KV file defines the layout and properties of your UI elements. You can then load this file in your Python script:
from kivy.app import App from kivy.uix.widget import Widget class HelloWorld(Widget): pass class HelloWorldApp(App): def build(self): return HelloWorld() if __name__ == '__main__': HelloWorldApp().run()
Advanced Kivy Concepts
As you become more comfortable with Kivy, you can explore more advanced features:
- Layouts: Use BoxLayout, GridLayout, or FloatLayout to organize your widgets.
- Input Handling: Implement touch, mouse, and keyboard events.
- Graphics: Utilize Kivy’s graphics instructions for custom drawing.
- Animations: Create smooth animations using Kivy’s animation classes.
- Data Binding: Use Kivy’s event system for reactive programming.
Best Practices for Kivy Development
To ensure your Kivy applications are efficient and maintainable, consider these best practices:
- Separate Logic and Presentation: Use KV language for UI design and Python for application logic.
- Leverage Kivy Properties: Use Kivy’s observable properties for reactive programming.
- Optimize Performance: Use Kivy’s built-in profiling tools to identify and fix bottlenecks.
- Follow Kivy Conventions: Adhere to Kivy’s naming conventions and design patterns.
- Test Regularly: Implement unit tests and conduct thorough cross-platform testing.
Packaging and Distribution
Once your application is ready, you’ll want to distribute it to users. Kivy works well with PyInstaller, which can create standalone executables for different platforms:
- Install PyInstaller:
pip install pyinstaller
- Create a spec file for your app
- Use PyInstaller to create the executable
Remember to test your packaged application on each target platform to ensure compatibility.
Conclusion
Python and Kivy offer a powerful, flexible solution for developing cross-platform desktop applications. With its intuitive design, rich feature set, and active community, Kivy empowers developers to create sophisticated applications that work seamlessly across different operating systems.
By mastering Python and Kivy, you’ll be well-equipped to tackle the challenges of modern desktop application development, creating software that’s both powerful and accessible to users on any platform.